Session和Cookie#
约 390 个字 7 行代码 3 张图片 预计阅读时间 8 分钟
Session入门案例#
获取session相关信息
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--获取Session--%>
<%=request.getSession().getAttribute("s")%><br>
<%--Session存活时间查询--%>
<%="Session存活时间:"+request.getSession().getMaxInactiveInterval()+"秒"%><br>
<%--上次访问时间间隔--%>
<%="上次访问时间间隔:"+(System.currentTimeMillis()-request.getSession().getLastAccessedTime())/1000+"秒"%><br>
<a href="addSession">添加Session</a>
<a href="deleteSession">删除Session</a>
</body>
</html>
package com.luguosong;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @author luguosong
*/
@WebServlet("/addSession")
public class AddSession extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
/*
* 添加Session
* */
req.getSession().setAttribute("s","hello Session");
//重定向回JSP页面
resp.sendRedirect(req.getContextPath()+"/hello_session.jsp");
}
}
Java
package com.luguosong;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @author luguosong
*/
@WebServlet("/deleteSession")
public class DeleteSession extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
/*
* 删除session
* */
//req.getSession().removeAttribute("s");
//或者
req.getSession().invalidate();
//重定向回JSP页面
resp.sendRedirect(req.getContextPath() + "/hello_session.jsp");
}
}
Session原理图解#
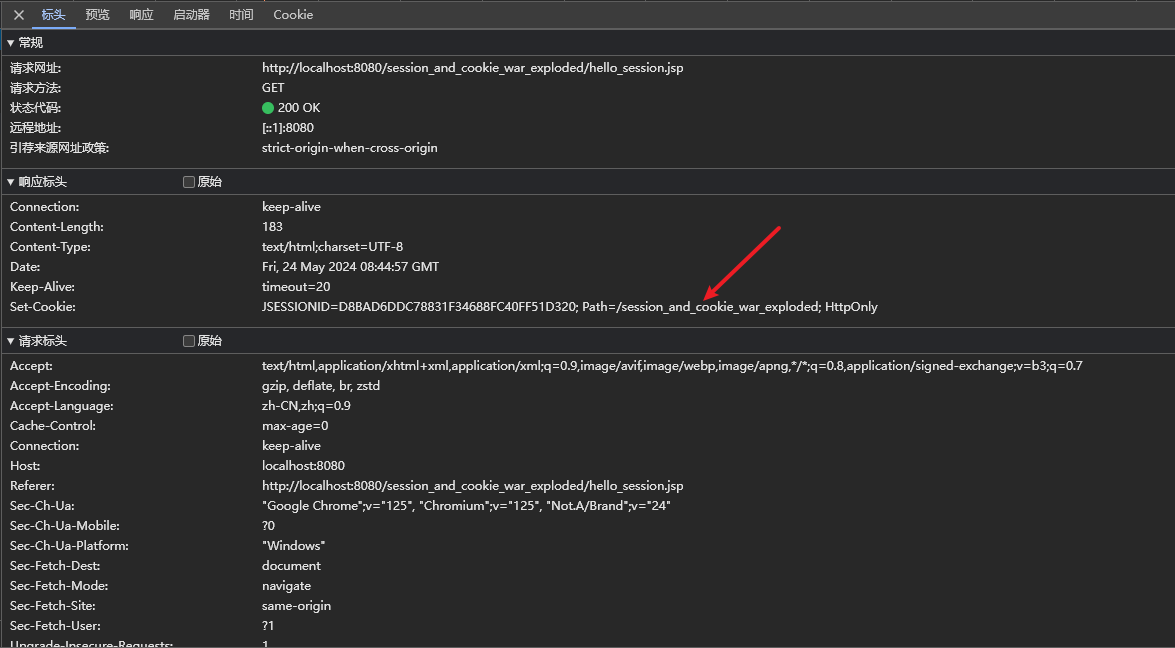
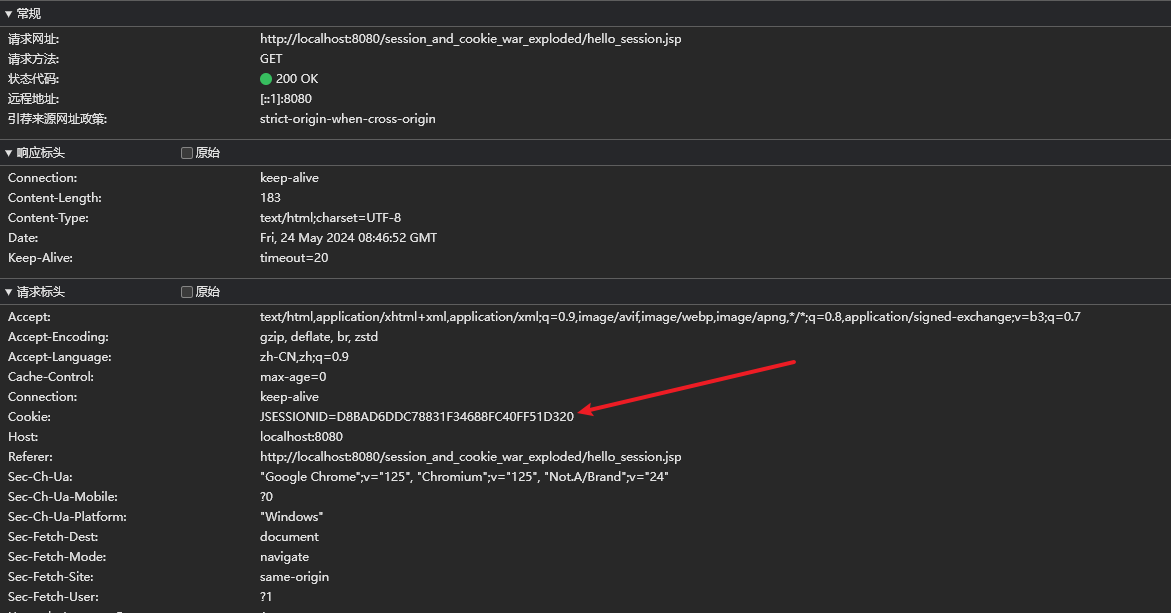
Session过期时间#
Session的默认超时时间是30分钟。
可以在Web.xml中进行自定义配置:
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="https://jakarta.ee/xml/ns/jakartaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/jakartaee https://jakarta.ee/xml/ns/jakartaee/web-app_6_0.xsd"
version="6.0">
<session-config>
<!--自定义Session超时时间为10分钟-->
<session-timeout>10</session-timeout>
</session-config>
</web-app>
Note
每次访问Servlet,Session的剩余超时时间都会刷新。
Cookie概述#
HTTP cookie
,简称cookie
,是浏览网站时由网络服务器创建并由网页浏览器存放在用户计算机或其他设备的小文本文件。
Cookie使Web服务器能在用户的设备存储状态信息
(如添加到在线商店购物车中的商品)或跟踪用户的浏览活动(如点击特定按钮、登录或记录历史)。
Cookie有效期#
- 不设置(默认,等价于小于0):运行
内存
中,浏览器关闭后Cookie消失。 - 大于0:保存在
硬盘
上,浏览器关闭Cookie仍然存在。 - 等于0:表示Cookie被删除,通常用这种方式删除浏览器上的同名Cookie。
请求何时携带Cookie#
默认路径
:如果不设置路径,Cookie的默认路径是创建该Cookie的Web应用的上下文路径
。路径匹配
:只有请求URL的路径与Cookie的路径
匹配时,浏览器才会在请求中发送该Cookie。
只有url在Cookie路径之下,request请求中才会包含对应的Cookie信息。
Cookie入门案例#
Java Server Page
<%@ page import="java.io.PrintWriter" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<div>
<%
PrintWriter writer = response.getWriter();
writer.println("当前Cookie:");
Cookie[] cookies = request.getCookies();
for (Cookie cookie : cookies) {
writer.println(cookie.getName());
writer.println(cookie.getValue() + "; ");
}
%>
</div>
<a href="addCookie">添加Cookie</a>
<a href="deleteCookie">清除Cookie</a>
</body>
</html>
Java
package com.luguosong;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.Cookie;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @author luguosong
*/
@WebServlet("/addCookie")
public class AddCookie extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//创建Cookie
Cookie cookie = new Cookie("key1", "helloCookie");
//设置Cookie过期时间为10秒
cookie.setMaxAge(10);
// 您指定的目录中的所有页面以及该目录子目录中的所有页面都能看到 cookie。
cookie.setPath(req.getContextPath());
resp.addCookie(cookie);
resp.sendRedirect(req.getContextPath()+"/hello_cookie.jsp");
}
}
Java
package com.luguosong;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.Cookie;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* @author luguosong
*/
@WebServlet("/deleteCookie")
public class DeleteCookie extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Cookie cookie = new Cookie("key1", "");
//将有效期设置为0,等价于清除同名Cookie
cookie.setMaxAge(0);
resp.addCookie(cookie);
resp.sendRedirect(req.getContextPath()+"/hello_cookie.jsp");
}
}