JSP#
约 915 个字 55 行代码 4 张图片 预计阅读时间 19 分钟
设置网站欢迎页#
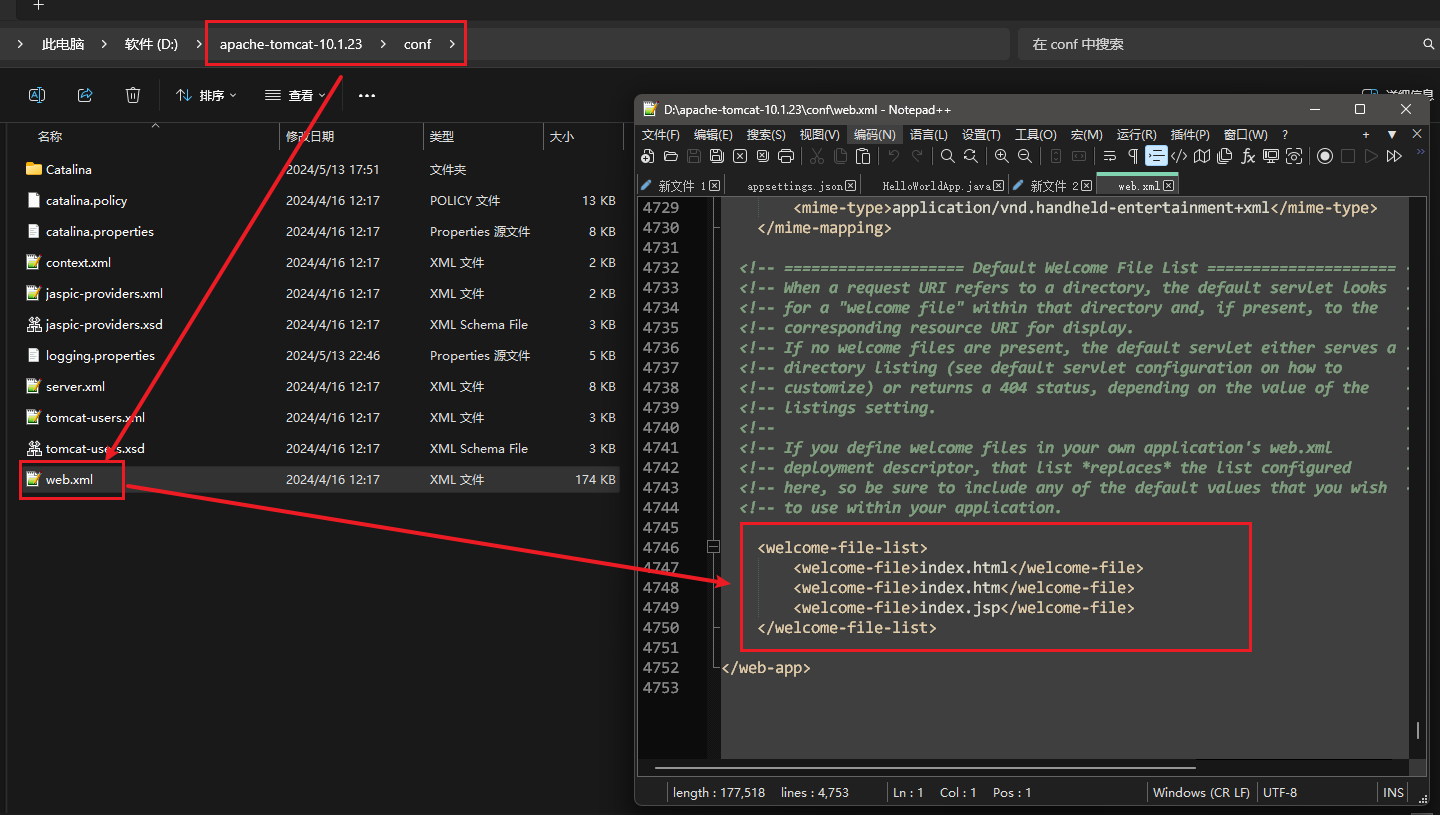
项目自定义配置欢迎页:
JSP概述#
JSP
(全称Jakarta Server Pages
,曾称为JavaServer Pages
)是由Sun微系统公司主导建立的一种动态网页技术标准。
JSP和Servlet
JSP文件在运行时会被其编译器转换成更原始的Servlet
程序码。JSP编译器
可以把JSP文件
转换成用Java程序码写的Servlet
,然后再由Java编译器
来编译成能快速执行的二进制机器码,也可以直接编译成二进制码。
JSP将Java程序码和特定变动内容嵌入到静态的页面中,实现以静态页面为模板,动态生成其中的部分内容。
JSP引入了被称为JSP动作
的XML标签,用来调用内置功能。
另外,可以创建JSP标签库
,然后像使用标准HTML或XML标签一样使用它们。标签库能增强功能和服务器性能,而且不受跨平台问题的限制。
Hello World#
.jsp
文件直接编写在WEB-INF目录
之外,可以直接访问。
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<br/>
<a href="hello-servlet">Hello Servlet</a>
你好
</body>
</html>
Tomcat会将.jsp
文件编译成Servlet
。
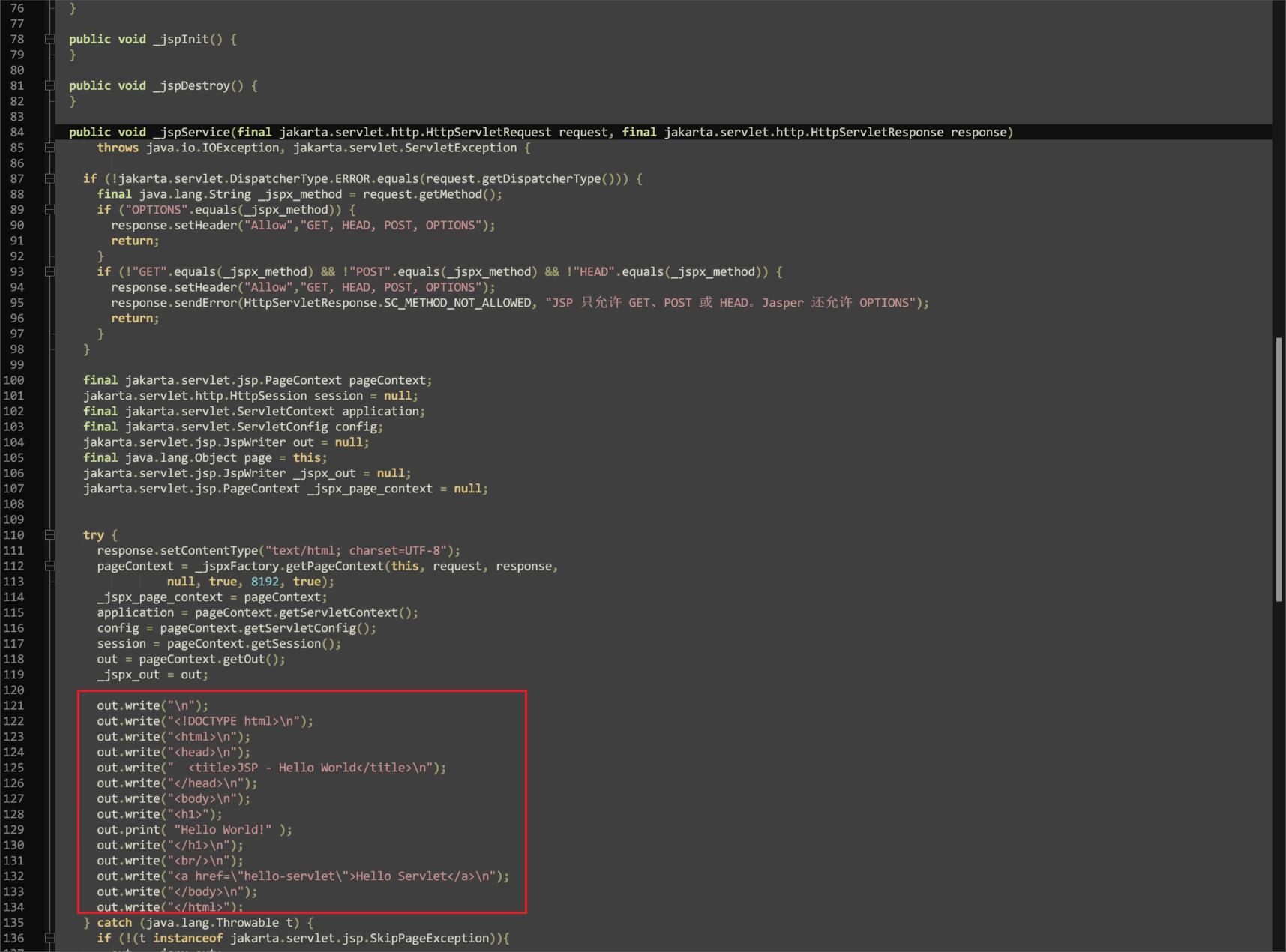
JSP中的注释#
JSP采用这种注释不会被翻译到Servlet
代码中去:
JSP中编写Java代码#
<% %>#
JSP中通过<% %>
添加Java代码,添加的代码会在service()方法
中执行。
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--在service()方法内部编写代码--%>
<%
System.out.println("在service方法内部执行");
%>
<%--等价于out.write(200+100);--%>
<%= 200+100 %>
<%--在service()方法之外编写代码--%>
<%--❗存在线程安全问题,一般不使用--%>
<%!
static {
System.out.println("在service方法外部执行");
}
%>
</body>
</html>
<%= %>#
<%= %>
等价于在service()方法
中执行out.write();
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--等价于out.write(200+100);--%>
<%= 200+100 %>
</body>
</html>
<%! %>#
<%! %>
中的代码会在service()方法
之外执行。
Warning
一般不常使用,因为Servlet在Tomcat中是单例的,会存在线程安全问题。
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%--在service()方法之外编写代码--%>
<%--❗存在线程安全问题,一般不使用--%>
<%!
static {
System.out.println("在service方法外部执行");
}
%>
</body>
</html>
JSP内置对象#
request对象#
使用request请求域
进行数据查询流程:
pageContext对象#
页面作用域,用于访问页面范围内的属性,并管理JSP页面的各种范围(如request、session、application)。
session对象#
会话作用域,等价于:
public class GetServletDemo extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
HttpSession session = req.getSession();
}
}
application对象#
应用作用域
response对象#
负责响应
out对象#
负责输出
exception对象#
用于处理JSP页面抛出的未处理异常。
config对象#
Servlet配置对象,等价于:
public class ServletConfigDemo extends GenericServlet {
@Override
public void service(ServletRequest req, ServletResponse res) throws ServletException, IOException {
ServletConfig config = getServletConfig();
}
}
page对象#
表示当前Servlet对象
指令#
指令
指导JSP翻译引擎如何工作。
// include指令,包含其他文件,在JSP中完成静态包含,很少用了。
<%include file="request_object.jsp"%>
// 引入标签库指令
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
// page指令
<%@ page contentType="text/html; charset=UTF-8" language="java" %>
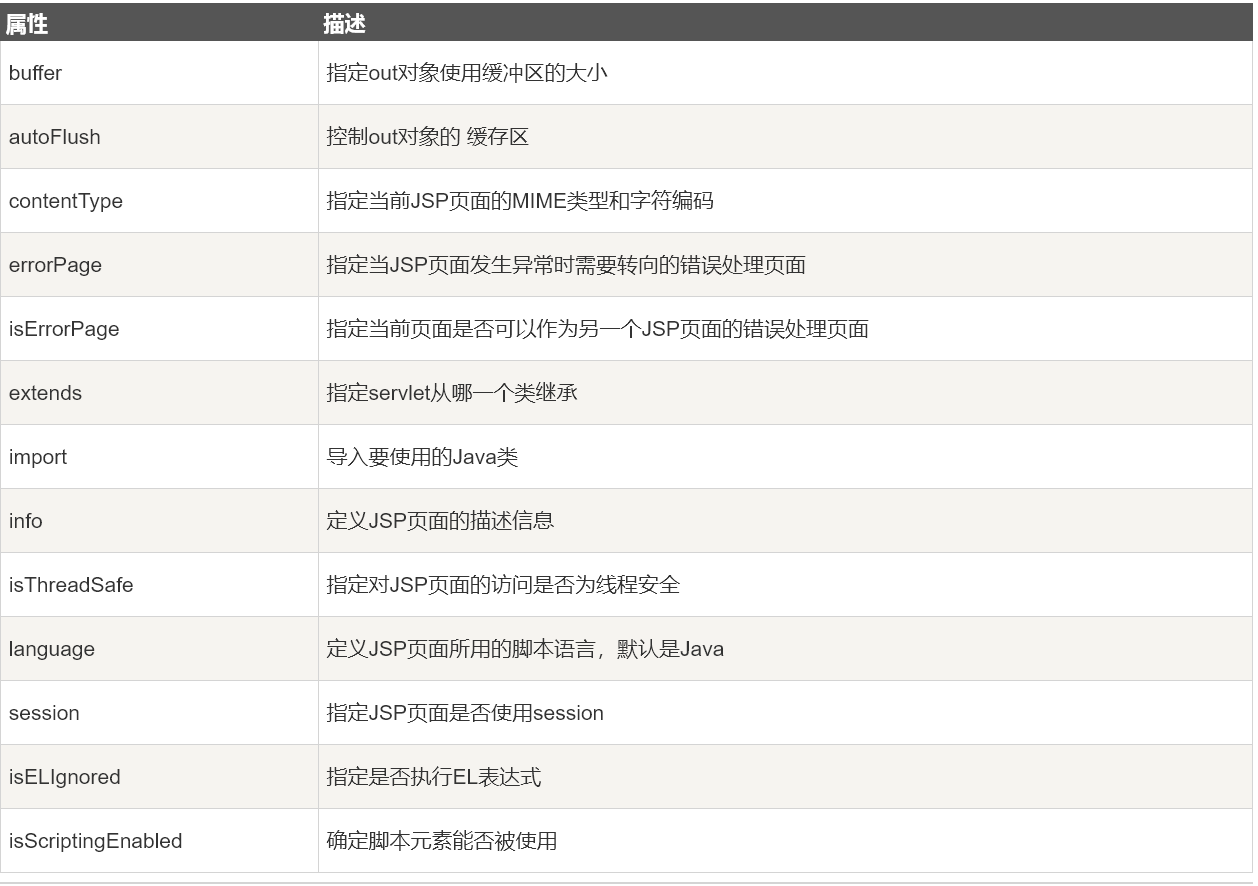
EL表达式#
EL表达式
(Expression Language)是一种用于在JavaServer Pages(JSP)和JavaServer Faces(JSF)等Java Web应用程序中嵌入表达式的语言。它允许开发人员在页面上直接访问和操作JavaBean组件的属性,以及调用Java方法,从而简化了在页面上处理数据的过程。 EL表达式通常用于动态地获取和显示数据,以及执行条件判断和迭代操作。
EL表达式
用于代替JSP中的Java代码,让JSP变得整洁。
获取域数据#
从PageContext域
、请求域
、会话域
、应用域
中获取数据
指定域范围#
El表达式优先级,域的范围越小,优先级越高
:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>EL表达式的优先级</title>
</head>
<body>
<%
System.out.println(111);
pageContext.setAttribute("key","pageContext");
request.setAttribute("key","request");
session.setAttribute("key","session");
application.setAttribute("key","ServletContext");
%>
<%--pageContext,默认情况下,从最小的返回读取--%>
${key}
<%--指定对应域获取--%>
<%--pageContext--%>
${pageScope.key}
<%--request--%>
${requestScope.key}
<%--session--%>
${sessionScope.key}
<%--ServletContext--%>
${applicationScope.key}
</body>
</html>
pageContext使用#
EL表达式
可以与pageContext对象
结合使用,获取内置对象。
Warning
EL表达式中是无法直接使用内置对象的。
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
${pageContext.request.contextPath}
<br><%--等价于--%>
<%=request.getContextPath()%>
</body>
</html>
param、paramValues使用#
param用于获取请求参数。
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
${param.username}
<br><%--等价于--%>
<%=request.getParameter("username")%>
<br>
${paramValues.hobby[0]}
${paramValues.hobby[1]}
${paramValues.hobby[2]}
<br><%--等价于--%>
<%=request.getParameterValues("hobby")%>
</body>
</html>
http://localhost:8080/jsp_el_war_exploded/param.jsp?username=%E5%BC%A0%E4%B8%89&hobby=%E6%8A%BD%E7%83%9F&hobby=%E5%96%9D%E9%85%92&hobby=%E7%83%AB%E5%A4%B4
initParam使用#
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="https://jakarta.ee/xml/ns/jakartaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/jakartaee https://jakarta.ee/xml/ns/jakartaee/web-app_6_0.xsd"
version="6.0">
<!--设置应用初始化参数-->
<context-param>
<param-name>username</param-name>
<param-value>李四</param-value>
</context-param>
</web-app>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>获取应用初始化参数</title>
</head>
<body>
${initParam.username}
<br><%--等价于--%>
<%=application.getInitParameter("username")%>
</body>
</html>
JSTL标签库#
JSTL
(JavaServer Pages Standard Tag Library)是一组用于简化JavaServer Pages(JSP)开发的标准标签。它提供了一套标签,用于执行常见的任务,如迭代集合、条件判断、格式化数据等。 JSTL使开发人员能够在JSP页面中使用标签而不是Java代码来实现常见的Web应用逻辑。
Maven依赖#
<dependencies>
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>jakarta.servlet.jsp.jstl</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>jakarta.servlet.jsp.jstl</groupId>
<artifactId>jakarta.servlet.jsp.jstl-api</artifactId>
<version>3.0.0</version>
</dependency>
</dependencies>
案例#
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%--引入核心标签库--%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
String[] fruits = {"Apple", "Banana", "Orange", "Grapes"};
request.setAttribute("fruits",fruits);
%>
<c:forEach var="fruit" items="${fruits}">
<div>${fruit}</div>
</c:forEach>
</body>
</html>